Updates to AutoConfiguration in Spring Boot 3
If you have a medium.com membership, I would appreciate it if you read this article on medium.com instead to support me~ Thank You! 🚀
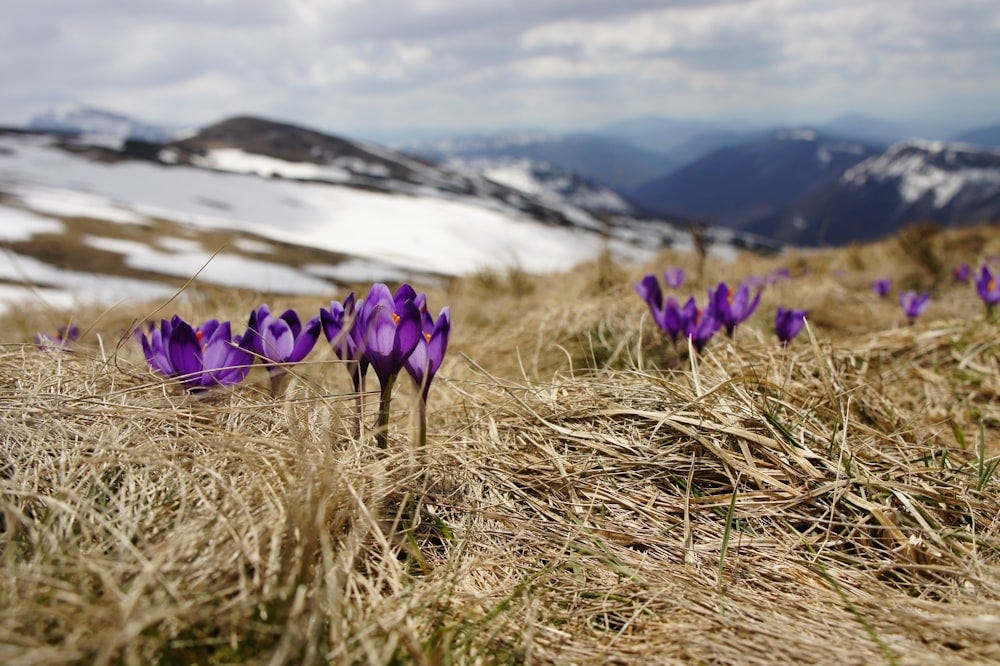
Intro
If you are using Auto-Configuration in Spring Boot, please take note that there is an update to the way you register your Auto-Configuration candidates. These changes were introduced in Spring Boot 2.7 and as of Spring Boot 3, the old way of configuring doesn’t seem to be working anymore. Refer to this GitHub issue for more information.
Read on to see the key changes and the minimal setup you need to get Auto-Configuration working in a custom Spring Boot starter library.
Background Context
project root
|- main-ms (depends on custom library)
|- custom-lib (starter library)
When working with Spring Boot multi-module project, you will typically have a microservice that depends on a few custom starter libraries. Below is how the pom.xml
or build.gradle.kts
would look like when the main microservice depends on the custom starter library.
<!-- pom.xml of the main-ms -->
<dependencies>
<dependency>
<groupId>com.example.library</groupId>
<artifactId>custom-lib</artifactId>
<version>0.0.1-SNAPSHOT</version>
</dependency>
</dependencies>
// build.gradle.kts (main-ms)
dependencies {
implementation(project(":custom-lib"))
}
For simplicity’s sake, I will be using @ComponentScan
as an example of when you would want to use Auto-Configuration.
project root
|- main-ms
|- src/main/kotlin/com/example/microservice
|- <your codes>
|- custom-lib (starter library)
|- src/main/kotlin/com/example/library
|- <your codes>
Notice that the package naming is different (com.example.microservice
vs com.example.library
). So for your project main-ms
to discover the beans configured in your custom-lib
, you will need to add this to your main-ms
:
package com.example.microservice
@SpringBootApplication(basePackages = "com.example.microservice")
@ComponentScan("com.example.library")
class Application
This works fine, but it requires the main-ms
to be aware of what’s the package name of the custom-lib
. An alternative is to make use of @ComponentScan
with an Auto-Configuration module within the custom-lib
to help the main-ms
automatically discover the beans. Let’s take a look at how to do that.
Auto-Configuration (Before Spring Boot 2.7)
This is how you would have done it before the update.
project root
|- custom-lib (starter library)
|- src/main
|- kotlin/com/example/library
|- config
|- LibraryAutoConfiguration.kts
|- resources
|- META-INF
|- spring.factories
Create the configuration below in your custom-lib
.
package com.example.library.config
@Configuration
@ComponentScan("com.example.library")
class LibraryAutoConfiguration
Then, register the configuration above as an Auto-Configuration candidate by adding it to the EnableAutoConfiguration
key in the resources/META-INF/spring.factories
file.
# Contents of spring.factories file
org.springframework.boot.autoconfigure.EnableAutoConfiguration=\
com.example.library.config.LibraryAutoConfiguration,\
... (any other configuration you want to add)
With these configurations, any microservices that depend on this library will know where to find the beans when importing. Next, let’s look at what are the new changes!
Auto-Configuration (After Spring Boot 2.7)
With the new updates, here’s how you should create your Auto-Configuration.
project root
|- custom-lib (starter library)
|- src/main
|- kotlin/com/example/library
|- config
|- LibraryAutoConfiguration.kts
|- resources
|- META-INF/spring
|- org.springframework.boot.autoconfigure.AutoConfiguration.imports
Create the configuration below in your custom-lib
. Instead of using @Configuration
, use the new annotation @AutoConfiguration
.
package com.example.library.config
@AutoConfiguration
@ComponentScan("com.example.library")
class LibraryAutoConfiguration
Then, when registering the configuration above as an Auto-Configuration candidate, instead of creating a spring.factories
, create a file named resources/META-INF/spring/org.springframework.boot.autoconfigure.AutoConfiguration.imports
and adds your configuration inside.
# Contents of org.springframework.boot.autoconfigure.AutoConfiguration.imports
com.example.library.config.LibraryAutoConfiguration
... (any other configuration you want to add)
With these configurations, any microservices that depend on this library will know where to find the beans when importing.
Conclusion
The Auto Configuration updates were introduced in Spring Boot 2.7 and the old configuration doesn’t seem to work in Spring Boot 3. Basic changes include:
- Using
@AutoConfigure
instead of@Configure
annotation - Replacing
spring.factories
withorg.springframework.boot.autoconfigure.AutoConfiguration.imports
That’s it! For more information, refer to these super useful links, and credit to people who wrote them:
- https://www.baeldung.com/spring-boot-custom-auto-configuration
- https://github.com/spring-projects/spring-boot/wiki/Spring-Boot-2.7-Release-Notes#new-autoconfiguration-annotation
- https://github.com/spring-projects/spring-boot/issues/32566
Thank you for reading till the end! ☕
If you enjoyed this article and would like to support my work, feel free to buy me a coffee on Ko-fi. Your support helps me keep creating, and I truly appreciate it! 🙏